In this tutorial I will share how I built a like button with CSS and Javascript.
As a UI and web designer I decided to make Javascript like button to be minimalistic. It has the basic functionality: heart microinteraction and counter.
So, let’s go and see the project in action below ⬇️
Project: JS like button with counter
// create like button in javascript
See the Pen JS Like Button by jsSecrets (@jssecrets) on CodePen.
Table of Contents
Basic Working Principle
What is Like Button in a nutshell?
It is a toggle mechanism which has two states: liked and not liked.
In the real life a light bulb switch resembles the like button’s working principle. It has two states ON and OFF.
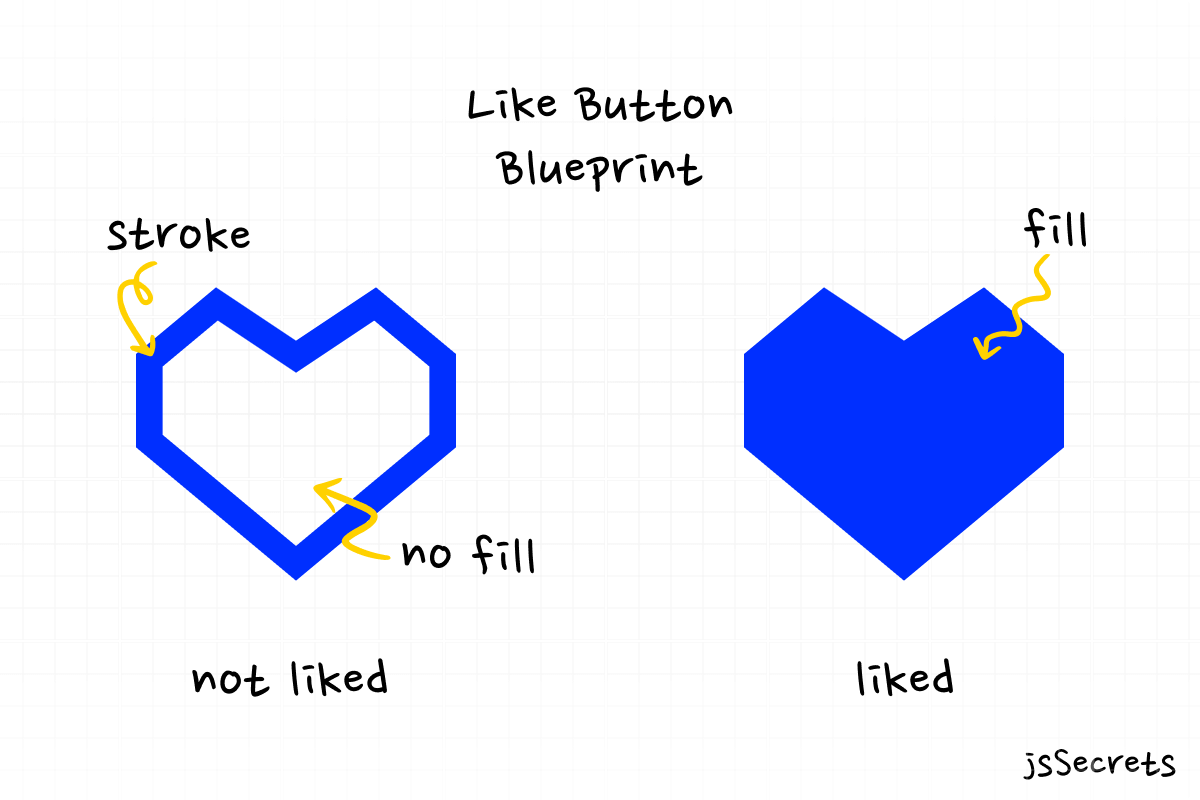
Working Principle
In the ordinary case we have an icon that may look like ? (thumbs up – YouTube) or ❤️ (heart – Instagram). In the “OFF” state the icon has a stroke, but no fill. In the “ON” state it has fill too.
Web designer may set, for instance, CSS class .liked
that will add the fill to the icon.
And in JS set the toggle function to switch between two states.
Core concept
// like and dislike button code in javascript
Let’s start with core concept of how Javascript like button works.
1️⃣ HTML
I have <svg>
heart element and a <span>
with a number (counter).
2️⃣ CSS
<svg>
heart shape has the visible outline and fill: transparent
when it is not liked. When the like button is clicked fill property changes to fill: {COLOR}
.
3️⃣ Javascript
In JS script I’ve coded a toggle function.
In the beginning I declare the let isLiked
variable and set it to false
.
Inside of the function I check this variable. If it is false
, then I add .isLiked
class to the like button, increase the value of the counter variable and set isLiked
variable to true
.
The opposite actions are performed if isLiked
variable has a true
value.
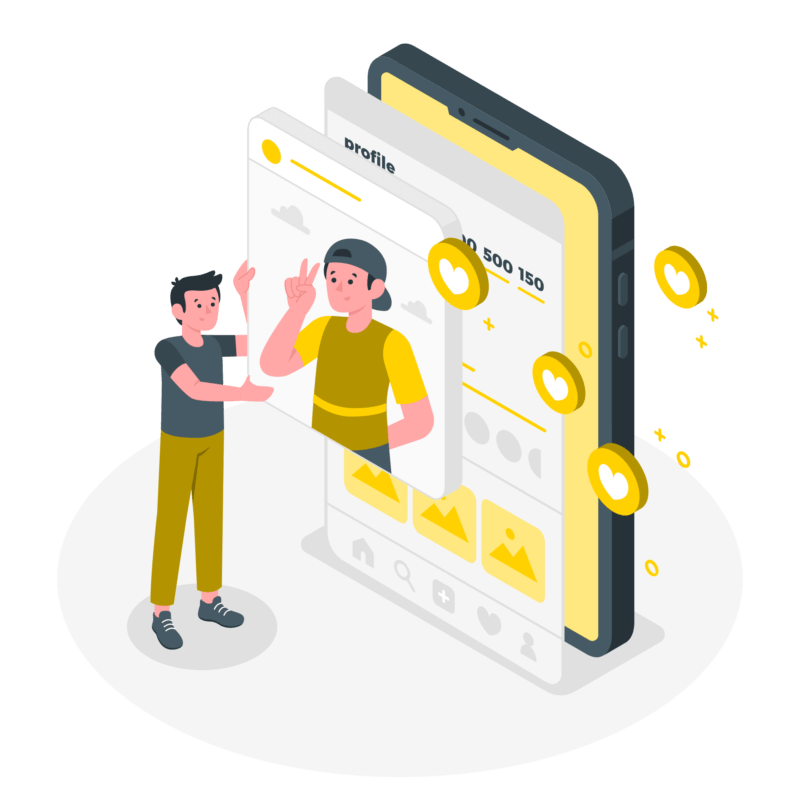
Code explanation
// code for like button in javascript
If you have understood the core concept of how the like button was built, the code will be easy to grasp.
HTML
// like and dislike button in javascript
<body>
<div class="block">
<!-- heart shape SVG -->
<svg
class="heart-icon"
width="106"
height="97"
viewBox="0 0 106 97"
fill="none"
xmlns="http://www.w3.org/2000/svg"
>
<!-- I am working with <path>, not the whole <svg> -->
<path
class="fill-color-shape"
fill-rule="evenodd"
clip-rule="evenodd"
d="M73.0406 3.04949C65.7359 2.94379 58.3559 5.38824 53.2483 12.3801C48.1271 5.39042 40.631 3.04941 33.4677 3.04941C18.2587 3.04941 3.04622 15.7081 3.04622 33.4698C3.04622 51.0995 14.3683 66.123 26.1679 76.6801C32.0812 81.9708 38.1493 86.1719 43.0557 89.0533C45.5086 90.4938 47.6791 91.6092 49.402 92.3672C50.2628 92.7459 51.0206 93.0393 51.6517 93.2395C52.2639 93.4336 52.8188 93.5607 53.2487 93.5607C53.6786 93.5607 54.2336 93.4336 54.8458 93.2395C55.4769 93.0393 56.2347 92.7459 57.0955 92.3672C58.8183 91.6092 60.9889 90.4938 63.4418 89.0533C68.3481 86.1719 74.4162 81.9708 80.3295 76.6801C92.1292 66.1229 103.451 51.0995 103.451 33.4698C103.451 15.6993 88.2313 3.26928 73.0406 3.04949Z"
/>
<path
d="M53.2483 12.3801L51.2316 13.8577C51.7029 14.5009 52.4527 14.8807 53.25 14.8801C54.0474 14.8796 54.7966 14.4987 55.267 13.8548L53.2483 12.3801ZM73.0406 3.04949L73.0767 0.549748L73.0406 3.04949ZM26.1679 76.6801L24.501 78.5433L24.501 78.5433L26.1679 76.6801ZM43.0557 89.0533L44.3217 86.8975L44.3217 86.8975L43.0557 89.0533ZM49.402 92.3672L50.4088 90.0789L50.4088 90.0789L49.402 92.3672ZM51.6517 93.2395L52.4075 90.8565L52.4074 90.8565L51.6517 93.2395ZM54.8458 93.2395L54.09 90.8565L54.09 90.8565L54.8458 93.2395ZM57.0955 92.3672L58.1023 94.6555L58.1023 94.6555L57.0955 92.3672ZM63.4418 89.0533L62.1757 86.8975L62.1757 86.8975L63.4418 89.0533ZM80.3295 76.6801L81.9965 78.5433L81.9965 78.5433L80.3295 76.6801ZM55.267 13.8548C59.7836 7.67202 66.2981 5.45219 73.0044 5.54923L73.0767 0.549748C65.1736 0.435397 56.9283 3.10445 51.2295 10.9054L55.267 13.8548ZM33.4677 5.54941C40.0922 5.54941 46.7154 7.69369 51.2316 13.8577L55.2649 10.9026C49.5387 3.08715 41.1697 0.549414 33.4677 0.549414V5.54941ZM5.54622 33.4698C5.54622 17.2225 19.5018 5.54941 33.4677 5.54941V0.549414C17.0157 0.549414 0.546219 14.1936 0.546219 33.4698H5.54622ZM27.8349 74.817C16.2174 64.4228 5.54622 50.0268 5.54622 33.4698H0.546219C0.546219 52.1722 12.5192 67.8231 24.501 78.5433L27.8349 74.817ZM44.3217 86.8975C39.5433 84.0912 33.6121 79.9859 27.8349 74.817L24.501 78.5433C30.5503 83.9556 36.7554 88.2525 41.7897 91.209L44.3217 86.8975ZM50.4088 90.0789C48.7945 89.3686 46.7116 88.3011 44.3217 86.8975L41.7897 91.209C44.3055 92.6865 46.5638 93.8497 48.3951 94.6555L50.4088 90.0789ZM52.4074 90.8565C51.8878 90.6917 51.2173 90.4346 50.4088 90.0789L48.3951 94.6555C49.3082 95.0572 50.1534 95.387 50.8959 95.6225L52.4074 90.8565ZM53.2487 91.0607C53.2633 91.0607 53.1984 91.0609 53.0284 91.0254C52.867 90.9918 52.6608 90.9368 52.4075 90.8565L50.8959 95.6225C51.5581 95.8325 52.417 96.0607 53.2487 96.0607V91.0607ZM54.09 90.8565C53.8367 90.9368 53.6305 90.9918 53.4691 91.0254C53.299 91.0609 53.2341 91.0607 53.2487 91.0607V96.0607C54.0805 96.0607 54.9393 95.8325 55.6016 95.6225L54.09 90.8565ZM56.0887 90.0789C55.2801 90.4346 54.6096 90.6917 54.09 90.8565L55.6016 95.6225C56.3441 95.387 57.1893 95.0572 58.1023 94.6555L56.0887 90.0789ZM62.1757 86.8975C59.7858 88.3011 57.703 89.3686 56.0887 90.0789L58.1023 94.6555C59.9337 93.8497 62.1919 92.6865 64.7078 91.209L62.1757 86.8975ZM78.6626 74.817C72.8853 79.9859 66.9542 84.0913 62.1757 86.8975L64.7078 91.209C69.742 88.2525 75.9471 83.9556 81.9965 78.5433L78.6626 74.817ZM100.951 33.4698C100.951 50.0268 90.2801 64.4228 78.6626 74.817L81.9965 78.5433C93.9783 67.8231 105.951 52.1722 105.951 33.4698H100.951ZM73.0044 5.54923C87.0131 5.75192 100.951 17.2432 100.951 33.4698H105.951C105.951 14.1555 89.4496 0.78665 73.0767 0.549748L73.0044 5.54923Z"
fill="#e74c3c"
/>
</svg>
<!-- counter -->
<span class="number-of-likes">6</span>
</div>
</body>
<svg>
acts like a container for its internal elements like <path>
, <circle>
, etc. Imagine that <svg>
is canvas and child elements are objects to interact with. Thinking in this paradigm will help you create many cool interactions.
CSS
// like button code in javascript
/*basic reset*/
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: "Urbanist", sans-serif;
}
body {
height: 100vh;
background: radial-gradient(circle, rgb(255, 255, 255) 70%, rgb(243, 243, 243) 100%);
position: relative;
}
.block {
width: 320px;
height: 160px;
background: white;
border-radius: 9px;
box-shadow: 0 10px 25px rgba(124, 130, 141, 0.2);
cursor: pointer;
transition: all 0.25s ease-out;
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
}
@media (max-width: 575.9px) {
.block {
width: 300px;
}
}
.block .heart-icon {
user-select: none;
position: absolute;
left: 20%;
top: 53%;
transform: translate(-20%, -50%);
fill: transparent;
transition: transform 0.25s ease-out;
transform-origin: 50% 50%;
}
/* initial state of the like heart button */
.block .heart-icon .fill-color-shape {
fill: transparent;
}
.block .heart-icon.isLiked {
filter: drop-shadow(0 3px 5px #c44133);
}
/* "liked" state of the like heart button */
.block .heart-icon.isLiked .fill-color-shape {
transition: fill 0.5s linear;
fill: #e74c3c;
}
.block .heart-icon:hover {
transform: translate(-20%, calc(-50% - 3px)) scale(1.03);
}
.block .number-of-likes {
font-size: 100px;
user-select: none;
position: absolute;
left: 80%;
top: 53%;
transform: translate(-80%, -50%);
color: #292d34;
}
Javascript
// like button using javascript
// Variables
const likeBtn = document.querySelector('.heart-icon');
const numberOfLikesElement = document.querySelector('.number-of-likes');
// get the value from the HTML element
let numberOfLikes = Number.parseInt(numberOfLikesElement.textContent, 10);
let isLiked = false;
// Functions
const likeClick = () => {
// if the like button hasn't been clicked
if (!isLiked) {
likeBtn.classList.add('isLiked');
numberOfLikes++;
numberOfLikesElement.textContent = numberOfLikes;
isLiked = !isLiked;
}
// if the like button has been clicked
else {
likeBtn.classList.remove('isLiked');
numberOfLikes--;
numberOfLikesElement.textContent = numberOfLikes;
isLiked = !isLiked;
}
};
// Event Listeners
likeBtn.addEventListener('click', likeClick);
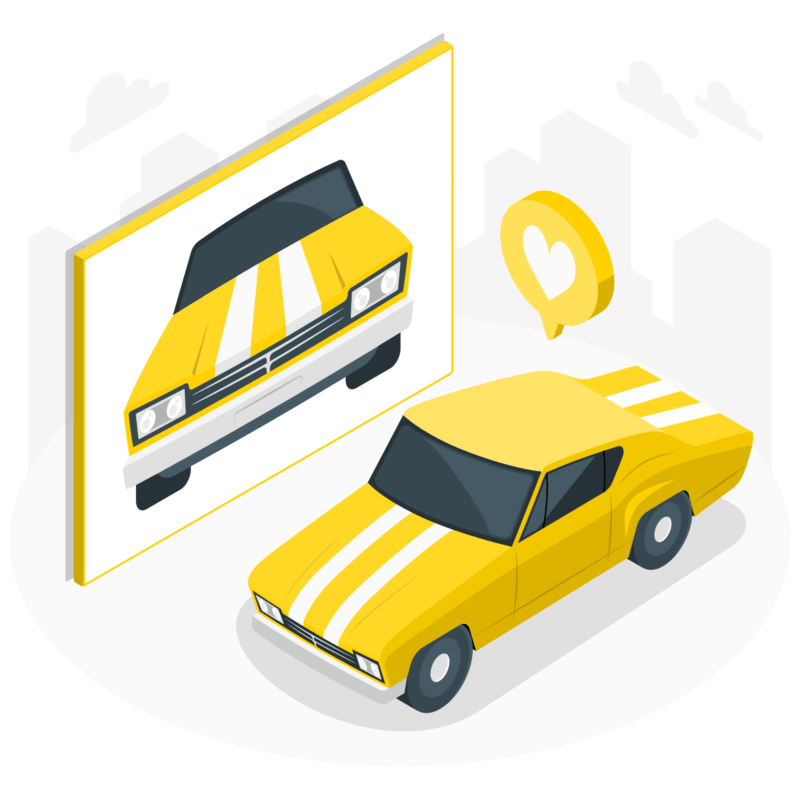
Project Ideas
// like button in js
1️⃣ Add an animation on click. Youtube has this animation effect. When the user clicks the like button small visual elements appear around it.
Frequently Asked Questions
// how to create a like button in html
1️⃣ Who invented a Like Button?
Justin Rosenstein, a former Facebook engineer built a prototype of Facebook’s “like” button at one of Facebook’s Hackathons.
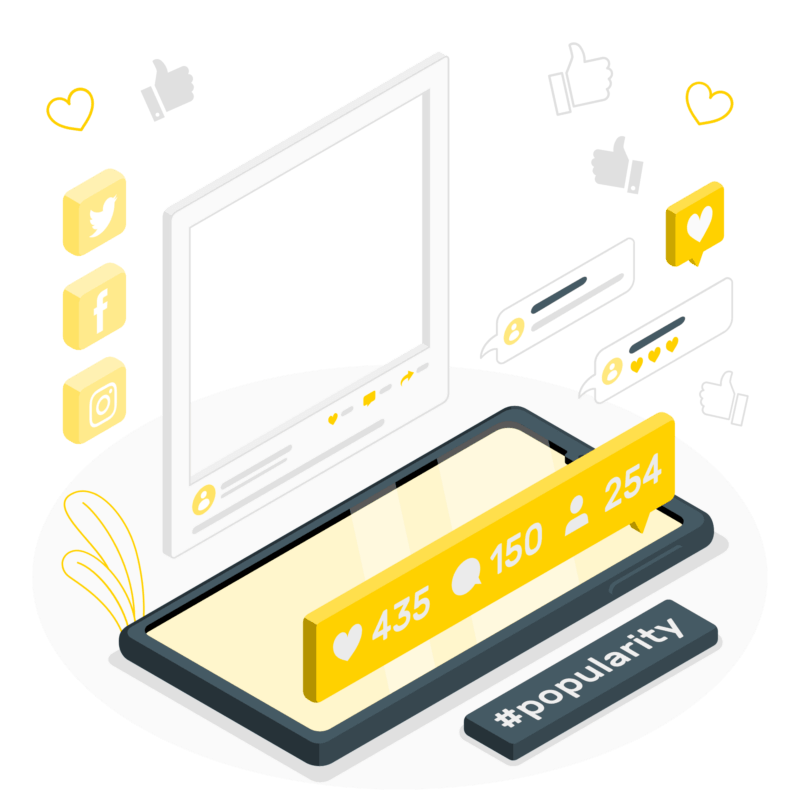
What to do next?
You can explore Hamburger Menu CSS & JS animation project.
Resources
// create a like button javascript
1️⃣ jssecrets.com
2️⃣ Transport illustrations by Storyset
3️⃣ Social media illustrations by Storyset