In this tutorial I will share how I built Password Validation in JS.
The project turned out to be modern and action-packed.
There are five a password condition validators onInput
event:
- lowercase letters
- uppercase letters
- digits
- special symbols
- longer than 8 characters
Also I have a password strength checker bar. (it is optional element)
And everything is animated which also creates a cool vibe 😊
As a UI designer, I decided to style JS password strength checker in Neu Brutalism UI style.
So, let’s go and see the project in action below ⬇️
Project: Password Validation in JS
guide on password validation in js
See the Pen Interactive Password Validation by jsSecrets (@jssecrets) on CodePen.
Table of Contents
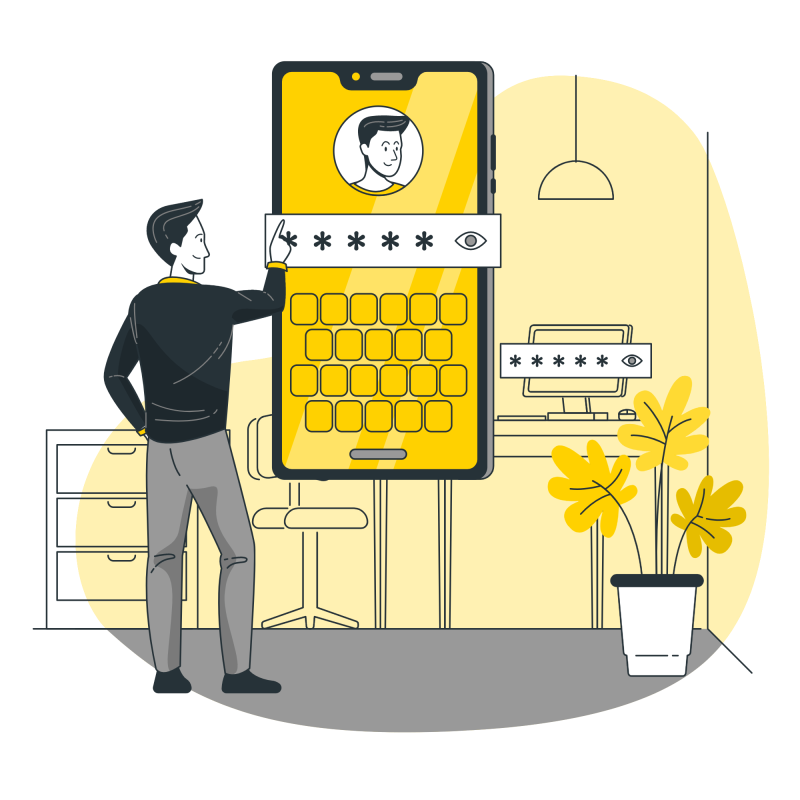
Basic Working Principle
guide on password validation in js
What is Password Validator / Input Validator in a nutshell?
guide on password validation in js
Sometimes we need user’s input to meet certain criteria. And input validation is one of the ways to achieve that.
One of the most common input validators we see in real life – is a bank card number validation. Major bank card input format is 16 digits. And each merchandise (Visa, MasterCard) has its hidden combinations.
That’s why if you try to enter some random bank card digits during the checkout – you will get an error.
Input validator won’t let you through.
And most of the times this is done with the help of Regular Expressions (regex).
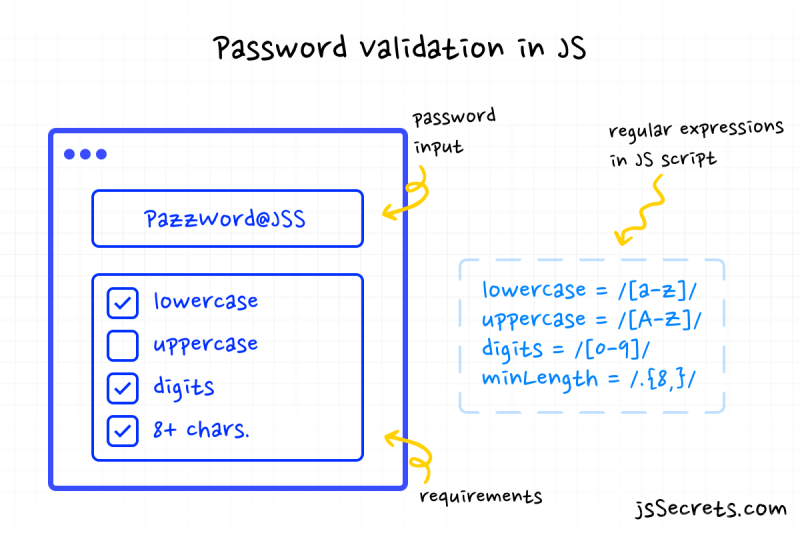
Working Principle
guide on password validation in js
Simplest Working Version (SWV)
guide on password regex javascript
The SWV of password validation in JS would probably be checking the input length. For example, password field must contain at least 8 characters.
Core concept
guide on password regex javascript
Let’s start with core concept of how JS password strength checker works.
1️⃣ HTML
guide on password regex javascript
In our project HTML contains:
- Password field (type=”text”) with 👁
- Strength bar (optional element)
- Requirements block
Checkmark icon for each requirement is SVG code. This is done for animation purposes.
2️⃣ CSS
guide on password regex javascript
CSS code for password validation in JS contains basic rules and several animations.
3️⃣ Javascript
guide on js password strength checker
The core of JS code is testPassword
function that checks user’s input against regular expressions.
The showHidePassword
function toggles input’s type: text and password.
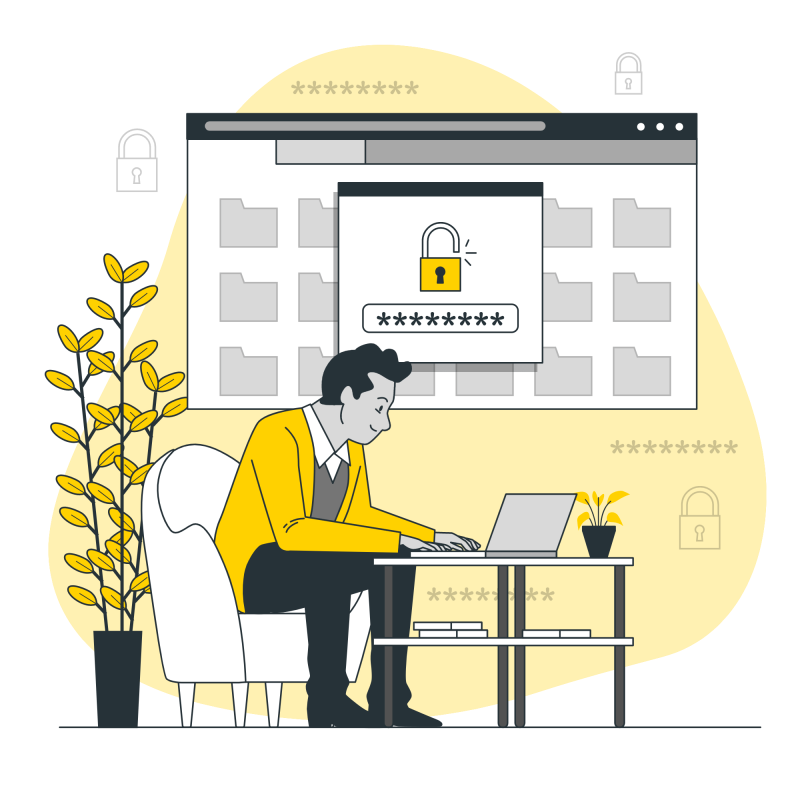
Code explanation
guide on js password strength checker
If you have understood the core concept and working principle of how to password validation in JS is built, the code will be easy to grasp.
HTML
guide on js password strength checker
<body>
<h1 class="title">Create your password</h1>
<div class="input-block">
<!-- input -->
<input class="primary" type="text" />
<!-- END input -->
<!-- eye -->
<div class="eye-container">
<div class="eye">
<div class="eye-face eye-face-front"></div>
<div class="eye-face eye-face-back"></div>
</div>
</div>
<!-- END eye -->
<!-- strength bar -->
<div class="strength-bar"></div>
<!-- END strength bar -->
</div>
<!-- requirements -->
<div class="req-block">
<ul class="req-list">
<li class="req lowercase">
<span class="checkmark-container">
<svg
class="checkmark"
xmlns="http://www.w3.org/2000/svg"
viewBox="0 0 26 26"
>
<circle class="circle" cx="13" cy="13" r="12.5" />
<path class="check" d="m5 13.807 4.773 4.84L21 7.353" />
</svg>
</span>
lowercase letters
</li>
<li class="req uppercase">
<span class="checkmark-container">
<svg
class="checkmark"
xmlns="http://www.w3.org/2000/svg"
viewBox="0 0 26 26"
>
<circle class="circle" cx="13" cy="13" r="12.5" />
<path class="check" d="m5 13.807 4.773 4.84L21 7.353" />
</svg>
</span>
uppercase letters
</li>
<li class="req digits">
<span class="checkmark-container">
<svg
class="checkmark"
xmlns="http://www.w3.org/2000/svg"
viewBox="0 0 26 26"
>
<circle class="circle" cx="13" cy="13" r="12.5" />
<path class="check" d="m5 13.807 4.773 4.84L21 7.353" />
</svg>
</span>
digits
</li>
<li class="req special-chars">
<span class="checkmark-container">
<svg
class="checkmark"
xmlns="http://www.w3.org/2000/svg"
viewBox="0 0 26 26"
>
<circle class="circle" cx="13" cy="13" r="12.5" />
<path class="check" d="m5 13.807 4.773 4.84L21 7.353" />
</svg>
</span>
special characters
</li>
<li class="req min-length">
<span class="checkmark-container">
<svg
class="checkmark"
xmlns="http://www.w3.org/2000/svg"
viewBox="0 0 26 26"
>
<circle class="circle" cx="13" cy="13" r="12.5" />
<path class="check" d="m5 13.807 4.773 4.84L21 7.353" />
</svg>
</span>
longer than 8 characters
</li>
</ul>
</div>
<!-- END requirements -->
</body>
CSS
guide on js password strength checker
/* basic reset */
*,
::before,
::after {
margin: 0;
padding: 0;
box-sizing: border-box;
}
ul {
list-style: none;
}
/* adding custom font */
@font-face {
font-family: "Gosha Sans";
src: url(../fonts/PPGoshaSans-Regular.otf);
font-weight: 400;
}
@font-face {
font-family: "Gosha Sans";
src: url(../fonts/PPGoshaSans-Bold.otf);
font-weight: 800;
}
body {
height: 100vh;
background: #ffc900;
position: relative;
display: flex;
justify-content: center;
align-items: center;
flex-direction: column;
overflow-x: hidden;
color: black;
font-family: "Gosha Sans", sans-serif;
font-weight: 400;
}
/* title */
.title {
font-size: 40px;
margin-bottom: 48px;
position: relative;
text-align: center;
}
@media (min-width: 575px) {
.title {
font-size: 64px;
}
}
@media (min-width: 768px) {
.title {
font-size: 100px;
}
}
/* input container for input field + eye, strength bar */
.input-block {
position: relative;
width: 220px;
height: 120px;
margin-bottom: 140px;
}
@media (min-width: 320px) {
.input-block {
width: 300px;
}
}
@media (min-width: 575px) {
.input-block {
width: 400px;
}
}
@media (min-width: 768px) {
.input-block {
width: 480px;
}
}
/* input field for password validation Javascript */
.input-block input.primary {
font-family: "Gosha Sans", sans-serif;
font-weight: 400;
font-size: 24px;
padding: 8px;
width: 100%;
height: auto;
border: 6px solid black;
box-shadow: 1px 1px black, 2px 2px black, 3px 3px black, 4px 4px black, 5px 5px black, 6px 6px black, 7px 7px black;
margin-bottom: 40px;
}
.input-block input.primary:focus {
outline: none;
}
@media (min-width: 575px) {
.input-block input.primary {
font-size: 40px;
padding: 12px;
height: 100%;
}
}
@media (min-width: 768px) {
.input-block input.primary {
font-size: 64px;
padding: 16px;
}
}
/* eye icon */
.input-block .eye-container {
position: absolute;
left: 95%;
top: 28px;
transform: translate(-95%, -50%);
width: 48px;
height: 48px;
perspective: 120px;
}
@media (min-width: 575px) {
.input-block .eye-container {
top: 50%;
transform: translate(-95%, -50%);
}
}
.input-block .eye-container .eye {
width: 100%;
height: 100%;
transition: transform 1s;
transform-style: preserve-3d;
cursor: pointer;
position: relative;
}
.input-block .eye-container .eye.invisible {
transform: rotateY(180deg);
}
.input-block .eye-container .eye .eye-face {
position: absolute;
width: 100%;
height: 100%;
backface-visibility: hidden;
background-position: center center;
background-size: cover;
background-repeat: no-repeat;
}
.input-block .eye-container .eye .eye-face-front {
background: url(../img/eye.svg);
}
.input-block .eye-container .eye .eye-face-back {
background: url(../img/eye-crossed.svg);
transform: rotateY(180deg);
}
/* requirements */
.req-block {
width: 220px;
background: #fff;
padding: 8px;
border: 6px solid black;
box-shadow: 1px 1px black, 2px 2px black, 3px 3px black, 4px 4px black;
}
@media (min-width: 320px) {
.req-block {
width: 300px;
padding: 12px;
}
}
@media (min-width: 575px) {
.req-block {
width: 400px;
padding: 16px;
}
}
@media (min-width: 768px) {
.req-block {
width: 480px;
padding: 24px;
}
}
.req-block .req {
font-size: 24px;
}
@media (min-width: 575px) {
.req-block .req {
font-size: 40px;
font-size: 24px;
}
}
@media (min-width: 768px) {
.req-block .req {
font-size: 64px;
font-size: 32px;
}
}
.req-block .req:not(:last-of-type) {
margin-bottom: 24px;
}
.req-block .req.validated {
color: #23a094;
}
/* checkmark */
.req-block .req .checkmark-container {
width: 40px;
height: 40px;
display: inline-block;
position: relative;
top: 8px;
}
.req-block .req .checkmark-container .checkmark {
fill-rule: evenodd;
clip-rule: evenodd;
stroke-linejoin: round;
stroke-miterlimit: 10;
box-shadow: inset 0px 0px 0px #23a094;
border-radius: 50%;
background-color: transparent;
pointer-events: none;
border: 3px solid black;
}
.req-block .req .checkmark-container .checkmark * {
fill: transparent;
stroke: transparent;
}
/* checkmark animation */
.req-block .req .checkmark-container .checkmark.animated {
animation: fill-checkmark 0.4s ease-in-out 0.4s forwards, scale 0.3s ease-in-out 0.9s both;
}
.req-block .req .checkmark-container .checkmark.animated .circle {
fill: none;
stroke-width: 2;
stroke-miterlimit: 10;
stroke: #23a094;
stroke-dasharray: 79;
stroke-dashoffset: 79;
animation: stroke 0.6s cubic-bezier(0.65, 0, 0.45, 1) forwards;
}
.req-block .req .checkmark-container .checkmark.animated .check {
fill: none;
fill-rule: nonzero;
stroke: #fff;
stroke-width: 2.5px;
stroke-dasharray: 23;
stroke-dashoffset: 23;
animation: stroke 0.3s cubic-bezier(0.65, 0, 0.45, 1) 0.8s forwards;
}
/* strength bar */
.strength-bar {
height: 32px;
width: 100%;
background: white;
position: relative;
border: 4px solid black;
box-shadow: 1px 1px black, 2px 2px black, 3px 3px black;
}
/* animated inner line of the bar */
.strength-bar::before {
content: "";
position: absolute;
left: 0;
height: 100%;
background: repeating-linear-gradient(45deg, #000000, #000000 6px, transparent 6px, transparent 12px);
/* "strength" variable is updated via JS */
width: calc(1% * var(--strength, 0));
transition: width 0.25s ease-in-out;
}
/* animations */
@keyframes stroke {
100% {
stroke-dashoffset: 0;
}
}
@keyframes scale {
0%, 100% {
transform: none;
}
50% {
transform: scale3d(1.1, 1.1, 1);
}
}
@keyframes fill-checkmark {
100% {
box-shadow: inset 0px 0px 0px 30px #23a094, 1px 1px black, 2px 2px black, 3px 3px black;
}
}
Javascript
guide on password checker js
// Variables
const passwordInput = document.querySelector('.primary');
const lowerCaseLabel = document.querySelector('.lowercase');
const upperCaseLabel = document.querySelector('.uppercase');
const digitsLabel = document.querySelector('.digits');
const specialCharsLabel = document.querySelector('.special-chars');
const minLengthLabel = document.querySelector('.min-length');
const strengthBar = document.querySelector('.strength-bar');
const eye = document.querySelector('.eye');
let strengthValue = 0;
// this object is used to create a loop for checking each regex
// and values of each key used for strength bar
const strengthParameters = {
lowerCase: false,
upperCase: false,
digits: false,
specialChars: false,
minLength: false,
};
// please pay attention to the naming format
// {name}Label | ex: lowerCaseLabel
// {name}RegEx | ex: lowerCaseRegEx
//// Regex
const lowerCaseRegEx = /[a-z]/;
const upperCaseRegEx = /[A-Z]/;
const digitsRegEx = /[0-9]/;
const specialCharsRegEx = /[^a-zA-Z0-9\s]/;
const minLengthRegEx = /.{8,}/;
// Functions
// 1. Test password
const testPassword = () => {
// loop through object
Object.keys(strengthParameters).forEach((parameter) => {
// if user's input passes test against regular expression
// then animation starts and color changes
// The eval() function evaluates JavaScript code
// represented as a string and returns its completion value.
// The source is parsed as a script.
if (eval(`${parameter}RegEx`).test(passwordInput.value)) {
eval(`${parameter}Label`).classList.add('validated');
// each "true" value adds 20% (100/5) to strength bar
strengthParameters[parameter] = true;
eval(`${parameter}Label`).children[0].children[0].classList.add(
'animated'
);
} else {
eval(`${parameter}Label`).classList.remove('validated');
strengthParameters[parameter] = false;
eval(`${parameter}Label`).children[0].children[0].classList.remove(
'animated'
);
}
// get number of "true" values in the object
// possible values from 0 to 5
// 20 * 0 = 0 (bar is empty)
// 20 * 5 = 100 (bar is full)
strengthValue =
20 * Object.values(strengthParameters).filter((value) => value).length;
// update "--strength" variable in CSS
strengthBar.style.setProperty('--strength', strengthValue);
/////////////////////////////////////////////////////
});
///////////////////////////////////////////////////
};
// 2. Show/hide password
const showHidePassword = (event) => {
event.currentTarget.classList.toggle('invisible');
// toggle between input type "text" and "password"
const type =
passwordInput.getAttribute('type') === 'password' ? 'text' : 'password';
passwordInput.setAttribute('type', type);
};
// Event Listeners
passwordInput.addEventListener('input', testPassword);
eye.addEventListener('click', showHidePassword);
Project Ideas
guide on password checker js
1️⃣ Credit card number validation
2️⃣ Validate Date of Birth
3️⃣ Validate Email (not with type=”email” 😉)
Frequently Asked Questions
guide on password checker js
1️⃣ What is password validation example?
You can see a great example of Password validation here: jssecrets.com/password-validation-in-js
2️⃣ How to check password validation in Javascript ?
The most common way is to check user’s input against regular expressions of your choice.
Examples: length, specific characters, etc.
3️⃣ How to put password validation in HTML?
HTML is a markup language and is not designed to handle password validation on its own. However, you can use JavaScript to add password validation to an HTML form.
Here is a great tutorial: jssecrets.com/password-validation-in-js
How to set password value in HTML?
You can set the default value of a password input field in HTML using the value
attribute. However, it is not recommended to pre-fill a password field with a default value, as it compromises the security of the password.
How to validate email and password in HTML?
HTML is a markup language and is not designed to handle email and password validation on its own. However, you can use JavaScript to add email and password validation to an HTML form.
What is verification and validation in password?
Verification checks whether the password meets the minimum security requirements, while validation checks whether the password entered by the user is correct and matches the password stored in the system. Both verification and validation are important aspects of password security, and they work together to ensure that passwords are both strong and secure.
How can I validate password in JavaScript?
You can see a great example of Password validation here: jssecrets.com/password-validation-in-js
How to use regex in JavaScript for password validation?
Here’s an example of how to use regular expressions (regex) in JavaScript for password validation:
function validatePassword(password) {
// Password must be at least 8 characters long and contain both letters and numbers
var regex = /^(?=.[a-zA-Z])(?=.[0-9])(?=.{8,})/;
return regex.test(password);
}
// Example usage
var password = "MyPassw0rd";
if (validatePassword(password)) {
console.log("Password is valid.");
} else {
console.log("Password is not valid.");
}
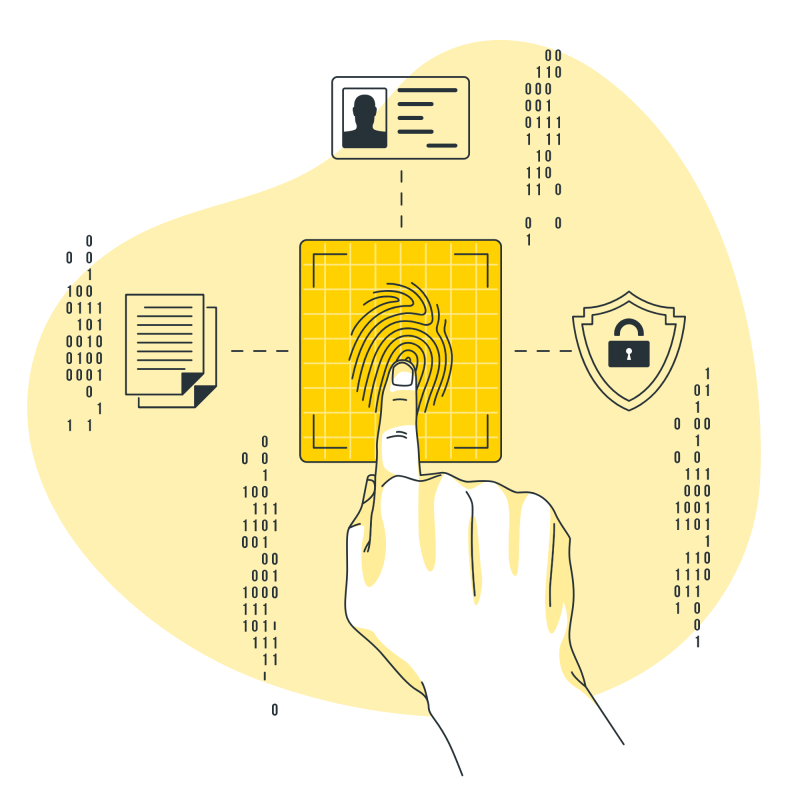
What to do next?
guide on confirm password validation Javascript
You can check out my Keyboard Input Filter project. It also uses JS regular expressions 😉
Resources
guide on password checker js
1️⃣ jssecrets.com
2️⃣ Javascript eval()
3️⃣ Data illustrations by Storyset
4️⃣ People illustrations by Storyset
5️⃣ Data illustrations by Storyset
6️⃣ Password Validation in JS at Codepen