Here is the general solution – a line of Javascript code that changes the background color:
DOM_ELEMENT.style.backgroundColor = COLOR
// change background color in JS
Here are a few examples of changing background color:
// example #1
document.body.style.backgroundColor = '#ff0000';
// in this example the DOM element is body (document.body)
// color is "#ff0000"
// this will change background color of body element to #ff0000 (red)
// example #2
document.querySelector('.your-css-selector').style.backgroundColor = 'green';
// in this example the DOM element is "your-css-selector" selector
// the color is green
Knowing this you can change background color of any DOM element.
As I frontend developer I decided to do a small project to master changing background color.
Project: Background color change in JS
// change background color in JS
Take a look and play with it clicking the paint image, which is acting like a button for changing background color.
See the Pen JS100::01-Change Background Color by jsSecrets (@jssecrets) on CodePen.
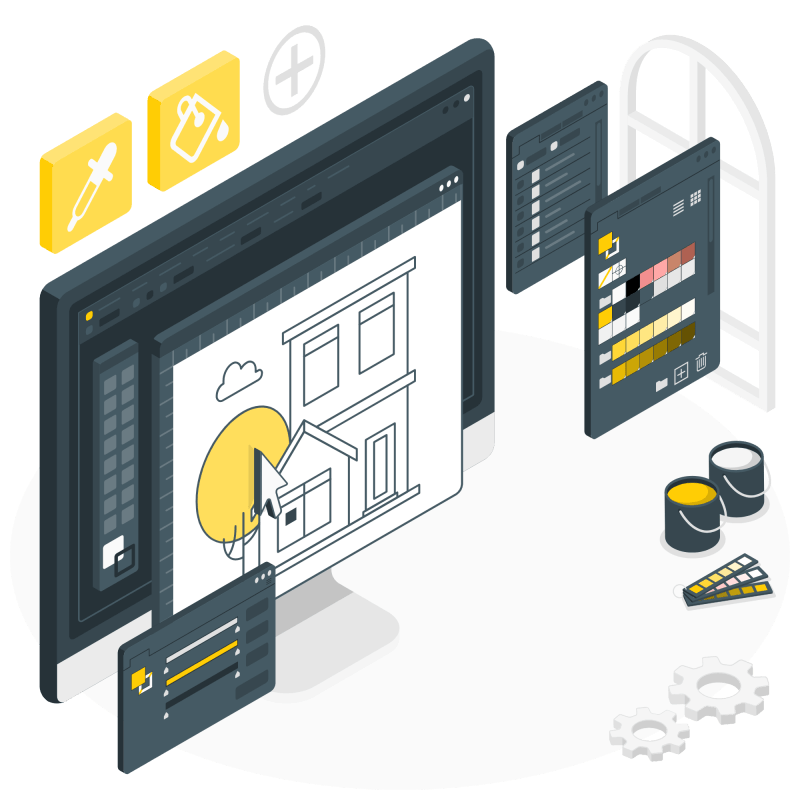
Code explanation
// change background color in JS
HTML
// change background color in JS
<body>
<div class="change-color-btn">
<img src="img/paints.png" />
</div>
</body>
Here I use only one <div>
that acts like a button. And inserted an image inside.
CSS
// change background color in JS
/* Basic reset */
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
body {
height: 100vh;
/* set initial background color body */
background-color: radial-gradient(
circle,
rgb(255, 255, 255) 70%,
rgb(243, 243, 243) 100%
);
/* Flexbox properties align button
horizontally and vertically centered */
display: flex;
justify-content: center;
align-items: center;
/* Transition properties make color
changing more smooth */
transition: all 750ms;
transition-delay: 0;
}
.change-color-btn {
width: 160px;
height: 160px;
background: white;
border-radius: 100%;
box-shadow: 0 10px 25px rgba(124, 130, 141, 0.2);
position: relative;
cursor: pointer;
transition: all 0.25s ease-out;
}
.change-color-btn:hover {
transform: translateY(-5px);
}
.change-color-btn img {
max-width: 70%;
/* Position the image in the very center
of the parent <div> block */
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
user-select: none;
}
JS
// change background color in JS
//// Variables
/* first off, I create an array and
fill it with a colors in the HEX format.
It can be any number of colors.
*/
const colors = ['#7b68ee', '#eb3dae', '#f7ce51', '#5bc5fa'];
/* next, create a "colorIndex" variable.
It will act as a box for an intermediate color.
*/
let colorIndex = 0;
/* lastly, select our button for changing color */
const button = document.querySelector('.change-color-btn');
//// Functions
/* our function that will be invoked on a 'click' event */
const changeColor = () => {
/* our DOM element is body (document.body) */
/* our color on the first click is the 'colors[0]' */
/* 'colors[0]' is the first element of 'colors' array */
document.body.style.backgroundColor = colors[colorIndex];
/* next we have a conditional (ternary) operator */
/* it means if the value of 'colorIndex' variable
less than length of the array, then increase it.
otherwise, set it to zero
*/
/* Hope you understand why we have 'length - 1' ? */
colorIndex < colors.length - 1 ? colorIndex++ : (colorIndex = 0);
/* The last line of code creates a loop
to change background infinitely */
};
//// Event Listeners
/* add event listener on button */
button.addEventListener('click', changeColor);
In this project I change background of body element, but it can done with any HTML element.
Let me also show you how to change background on any element with a custom function.
Custom function
/* our custom function that will change a background */
/* it accepts two parameters */
/* an element and a color */
const bgColorChange = (element, color) => {
element.style.backgroundColor = color;
};
/* function call */
/* our element is the '.box' selector element */
/* the color is yellow */
bgColorChange(document.querySelector('.box'), 'yellow');
Try this code and you will see the result 🙂.
I also want to share couple of ideas on projects where you can practice changing backgorund color with JS.
Project ideas
1️⃣ Change background with a random color
You can accomplish this in different ways. For instance, every time you click a button a random color is generated or generate an array of random colors.
2️⃣ Pick a background color
Even more ideas here, pick a color from a dropdown list or just create a color grid. More advanced javascripters may use a color wheel component.
Do you have any other idea?
Yes?
Share it in comments 🙂
What to do next?
You can explore some of my latest projects here.
Thanks for your blog, nice to read. Do not stop.
Thank you Mark! ?