In this tutorial I will share how I built Javascript Sidebar Menu.
Collapsible sidebar is one of the most used UI elements on websites nowadays, that’s why it’s important skill for a web designer to know how to build it.
As a UI designer, I decided to style sidebar in Modern Minimal UI style 😍.
So, let’s go and see the project in action below ⬇️
Project: Javascript Sidebar Menu
// javascript sidebar menu
See the Pen Sidebar Javascript by jsSecrets (@jssecrets) on CodePen.
Table of Contents
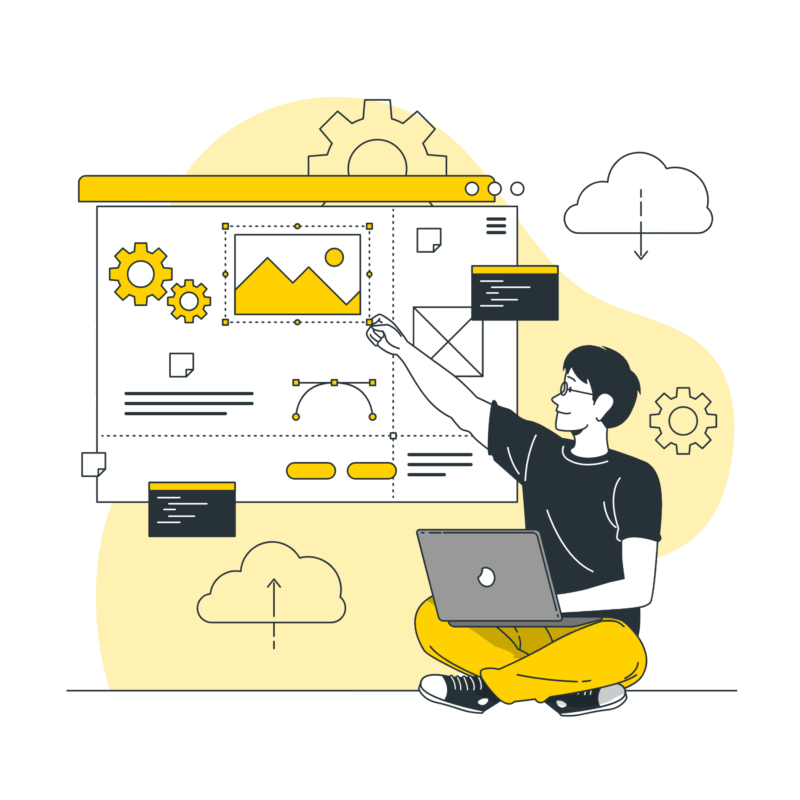
Basic Working Principle
// javascript sidebar menu
What is collapsible Sidebar in a nutshell?
// javascript sidebar menu
Sidebar panel is an element that is mostly used as a container for website/app menu or navigation.
It is hidden by default, usually, behind the left or right side of the screen. When the trigger is clicked/touched sidebar appears.
One of the most popular usages os collapsible sidebar is the mobile menu on the website. When screen width becomes too narrow, it helps properly display horizontal navigation. Navigation disappears and sidebar appears.
Javascript Sidebar Menu Blueprint
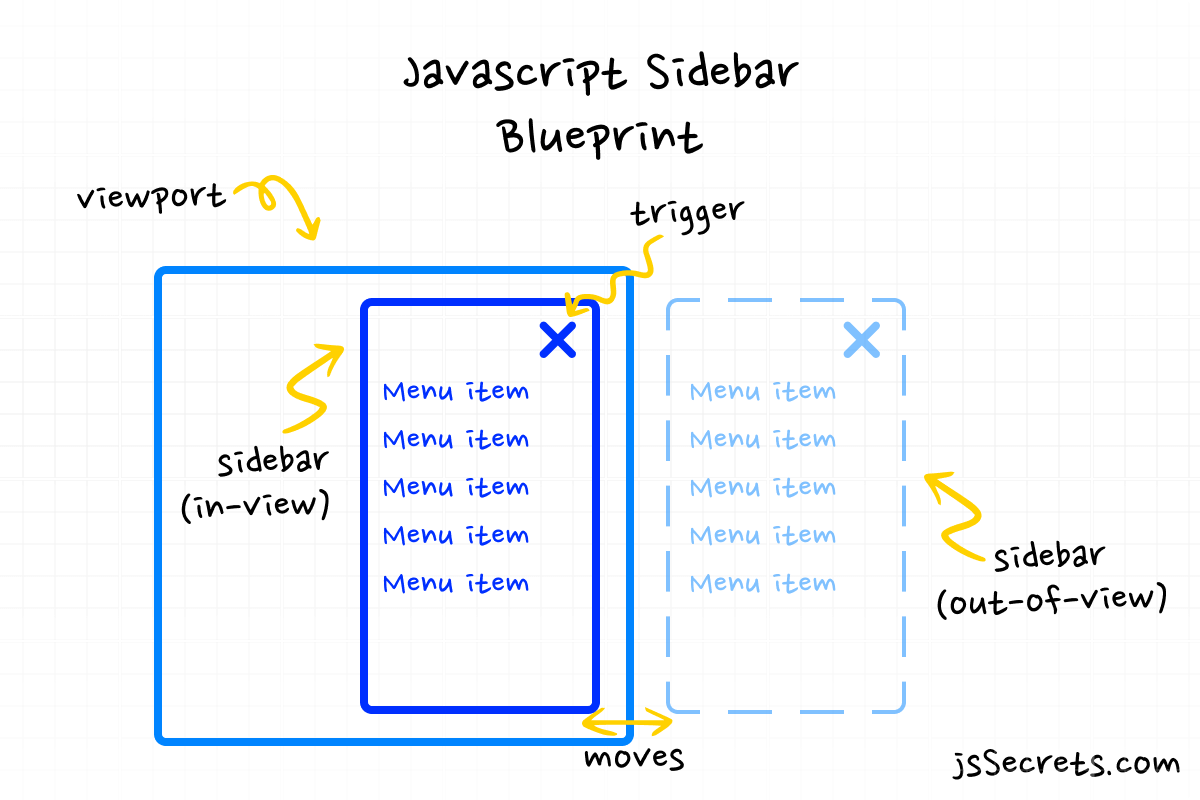
Working Principle
// css sidebar examples
Simplest Working Version (SWV)
// css sidebar examples
The SWV of JS sidebar menu is a container with those CSS properties:
height: 100%;
position: fixed;
top: 0;
right: 0; /*or left:0;*/
width: 320px;
z-index: 500;
It means that sidebar is attached to the right side of the screen and it is on top of other elements, occupying 100% of the screen height.
And we also need a trigger button to show/hide a sidebar.
SWV = sidebar panel + trigger button 😊
Core concept
// css sidebar examples
Let’s start with core concept of how all of this works.
1️⃣ HTML
// css sidebar examples
.sidebar
contains <img>
logo and <ul>
list with <li>
items. And also .trigger
element.
2️⃣ CSS
// sidebar website examples
As explained above sidebar is fixed to the right side of the screen.
To remove it from view I use:
transform: translateX(100%)
– out of view.
When sidebar gets the .show
class it animates into view i.e.
transform: translateX(0)
And transition: transform 0.5s
property makes animation smooth.
3️⃣ Javascript
// sidebar website examples
JS has only one function that toggles the .show
class.
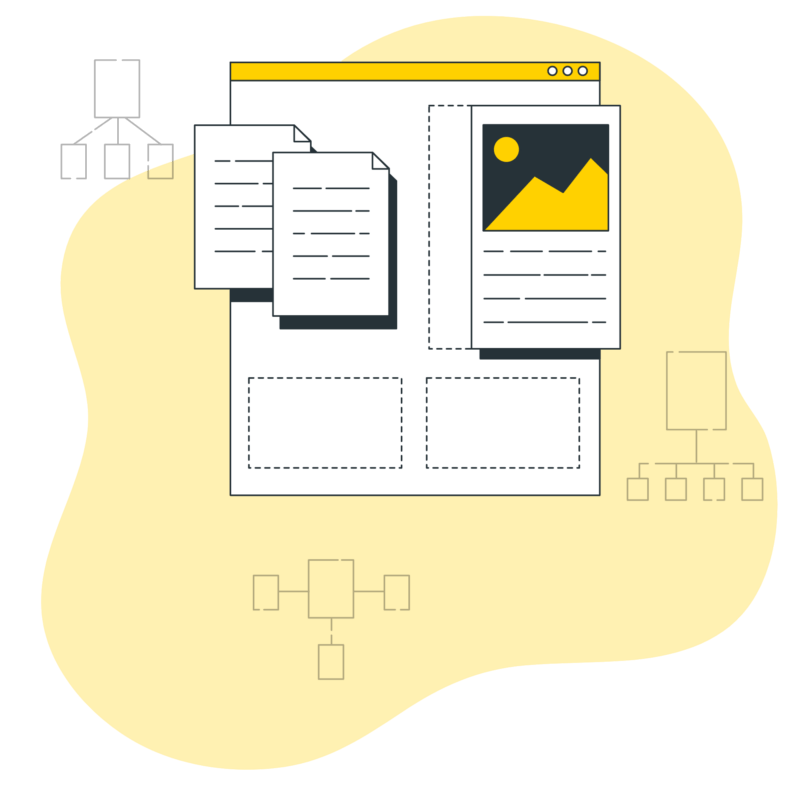
Code explanation
// sidebar website examples
If you have understood the core concept and working principle of how to javascript sidebar menu is built, the code will be easy to grasp.
HTML
// sidebar website examples
<body>
<!--trigger button-->
<div class="nav">
<div class="trigger"><span></span></div>
</div>
<!--sidebar button-->
<div class="sidebar">
<div class="content">
<a class="logo">
<img src="https://1674814792-20d01e3358a6b87a.wp-transfer.sgvps.net/wp-content/uploads/2022/07/logo.png" />
</a>
<ul class="menu">
<li>HTML</li>
<li>CSS</li>
<li>JS</li>
<li>UI Design</li>
</ul>
</div>
</div>
</body>
CSS
// sidebar javascript example
/*basic reset*/
*,
::before,
::after {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: "Poppins", sans-serif;
}
ul {
list-style: none;
}
body {
height: 100vh;
background: radial-gradient(circle, rgb(255, 255, 255) 70%, rgb(243, 243, 243) 100%);
position: relative;
overflow: hidden;
color: #292d34;
}
/*navigation for trigger*/
/*it is optional element*/
/*you can simply have <button> (for example)*/
.nav {
width: 100%;
height: 100px;
position: relative;
z-index: 1000;
}
/*trigger button*/
/*learn how to animate Burger Menu at the link below:*/
/*https://1674814792-20d01e3358a6b87a.wp-transfer.sgvps.net/how-to-build-hamburger-menu-css-animation/*/
.nav .trigger {
position: absolute;
top: 50%;
right: 24px;
transform: translate(0, -50%);
cursor: pointer;
display: inline-block;
width: 48px;
height: 30px;
}
.nav .trigger span {
position: relative;
width: 100%;
height: 6px;
transform: translateY(12px);
background-color: #292d34;
display: block;
border-radius: 9px;
}
.nav .trigger span::before, .nav .trigger span::after {
position: absolute;
content: "";
width: 100%;
height: 6px;
background-color: #292d34;
display: block;
border-radius: 9px;
}
.nav .trigger span::before {
transform: translateY(-12px);
}
.nav .trigger span::after {
transform: translateY(12px);
}
.trigger span {
transition: all 0.3s linear;
}
.trigger.active span {
background-color: transparent;
}
.trigger span:before {
animation: downAndTurnReverse 0.5s linear both;
}
.trigger.active span:before {
animation: downAndTurn 0.5s linear both;
}
.trigger span:after {
animation: upAndTurnReverse 0.5s linear both;
}
.trigger.active span:after {
animation: upAndTurn 0.5s linear both;
}
/*trigger animation*/
@keyframes downAndTurn {
0% {
transform: translateY(-12px) rotate(0deg);
}
50% {
transform: translateY(0px) rotate(0deg);
}
100% {
transform: translateY(0px) rotate(45deg);
}
}
@keyframes downAndTurnReverse {
0% {
transform: translateY(0) rotate(45deg);
}
50% {
transform: translateY(0px) rotate(0deg);
}
100% {
transform: translateY(-12px) rotate(0deg);
}
}
@keyframes upAndTurn {
0% {
transform: translateY(12px) rotate(0deg);
}
50% {
transform: translateY(0px) rotate(0deg);
}
100% {
transform: translateY(0px) rotate(-45deg);
}
}
@keyframes upAndTurnReverse {
0% {
transform: translateY(0px) rotate(-45deg);
}
50% {
transform: translateY(0px) rotate(0deg);
}
100% {
transform: translateY(12px) rotate(0deg);
}
}
/*sidebar*/
.sidebar {
position: fixed;
top: 0;
right: 0;
width: 320px;
max-width: 90%;
height: 100%;
color: #fff;
z-index: 500;
background: linear-gradient(45deg, rgb(245, 205, 81) 0%, rgb(247, 206, 81) 100%);
overflow-y: auto;
padding: 32px;
overflow-x: hidden;
transition: transform 0.5s;
/*to set sidebar out of view i.e. invisible state*/
transform: translateX(100%);
}
@media (max-width: 320px) {
.sidebar {
padding: 24px;
}
}
.sidebar .logo {
font-size: 32px;
display: block;
margin-bottom: 48px;
position: relative;
top: -2px;
transition: all 1s ease-out;
/*I moved the element to right for future animation*/
/*it will move from 150px to 0px*/
transform: translateX(150px);
}
.sidebar .logo img {
max-width: 160px;
user-select: none;
}
@media (max-width: 320px) {
.sidebar .logo img {
max-width: 120px;
}
}
.sidebar .menu li {
font-size: 24px;
color: #292d34;
cursor: pointer;
/*I moved the element to right for future animation*/
/*it will move from 150px to 0px*/
transform: translateX(150px);
}
@media (max-width: 320px) {
.sidebar .menu li {
font-size: 20px;
}
}
/*stagger animation*/
.sidebar .menu li:nth-of-type(1) {
transition: all 1.1s ease-out;
}
.sidebar .menu li:nth-of-type(2) {
transition: all 1.2s ease-out;
}
.sidebar .menu li:nth-of-type(3) {
transition: all 1.3s ease-out;
}
.sidebar .menu li:nth-of-type(4) {
transition: all 1.4s ease-out;
}
/*visible state */
.sidebar.show {
transform: translateX(0);
box-shadow: -10px 0px 35px rgba(124, 130, 141, 0.3);
}
.sidebar.show .logo {
transform: translateX(0);
}
.sidebar.show .menu li {
transform: translateX(0);
}
Javascript
// sidebar javascript example
// Variables
const trigger = document.querySelector('.trigger');
const sidebar = document.querySelector('.sidebar');
// Functions
const sidebarToggle = () => {
trigger.classList.toggle('active');
sidebar.classList.toggle('show');
};
// Event Listeners
trigger.addEventListener('click', sidebarToggle);
Project Ideas
// sidebar javascript example
1️⃣ Horizontal sidebar that animates from the top or bottom of the screen.
2️⃣ Sticky sidebar like you saw on documentation pages. (ex: Bootstrap)
Frequently Asked Questions
// sidebar javascript example
1️⃣ What is a sidebar?
Sidebar panel is an element that is mostly used as a container for website/app menu or navigation.
It is hidden by default, usually, behind the left or right side of the screen. When the trigger is clicked/touched sidebar appears.
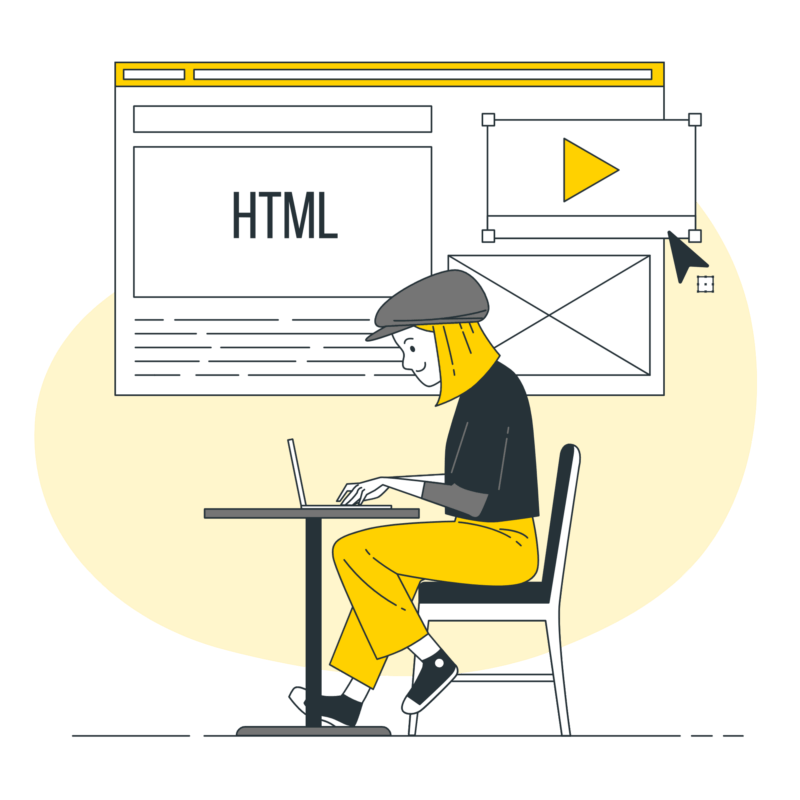
What to do next?
// sidebar javascript example
You can check out other JS tutorials:
2️⃣ Multi Level Menu
3️⃣ Accordion
Resources
// sidebar javascript example
1️⃣ jssecrets.com
2️⃣ Online illustrations by Storyset
3️⃣ Online illustrations by Storyset